In today’s digital world, organizing and managing contact information is crucial for both personal and professional reasons. Whether it’s keeping track of your friends’ phone numbers or organizing business contacts, a contact management system can help you stay organized and ensure that your contact information is always up-to-date.
A contact manager is a tool that helps store and manage contact details, such as names, phone numbers, email addresses, and other relevant data. While there are many commercially available contact management solutions, creating your own custom contact manager using Python can give you a better understanding of programming principles and enable you to tailor the system to your specific needs.
Why Build a Contact Manager with Python?
Python is an excellent choice for building a contact manager for several reasons:
- Simple and Readable Code: Python’s easy-to-understand syntax and clear structure make it an ideal language for beginners and intermediate programmers.
- Extensive Libraries: Python offers a wide array of libraries that allow you to work with databases, JSON, CSV files, and even build graphical user interfaces (GUIs) for your project.
- Cross-Platform Compatibility: Python is cross-platform, meaning that your contact manager can run on Windows, MacOS, or Linux without any major adjustments.
- Scalability: Python provides the flexibility to scale your project, so whether you’re managing a handful of contacts or thousands of them, Python can handle your needs.
Building Your Own Contact Manager in Python
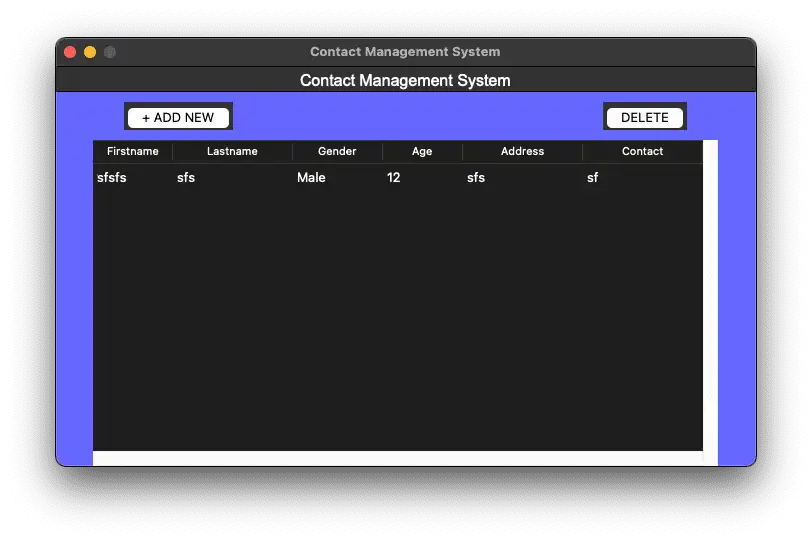
Now that we’ve established why Python is a great tool for creating a contact manager, let’s walk through the process of building one.
Step 1: Setting Up Your Project
To start, you will need to organize your project. Create a directory for your project and set up your Python file and any necessary storage files. For this project, we’ll use JSON files to store contact data, but you can opt for other databases like SQLite or MySQL if your application requires more complex data handling.
Here’s how to get started:
- Create a folder for your project, e.g., contact_manager.
- Inside that folder, create a Python file, e.g., contact_manager.py.
- Create an empty JSON file to hold your contacts, e.g., contacts.json.
Step 2: Designing the Contact Class
In object-oriented programming (OOP), a class is a blueprint for creating objects. We’ll design a Contact class to represent each contact in our system. Each contact will have attributes like name, phone number, and email.
python
CopyEdit
class Contact:
def __init__(self, name, phone, email):
self.name = name
self.phone = phone
self.email = email
def __str__(self):
return f”Name: {self.name}, Phone: {self.phone}, Email: {self.email}”
- The __init__ method initializes a contact with its attributes: name, phone, and email.
- The __str__ method is used to print the contact details in a readable format when we display or log contact information.
Step 3: Functions for Managing Contacts
To make the contact manager functional, we need to implement a series of functions that allow users to:
- Add contacts
- View all contacts
- Delete a contact
- Search for a contact
We’ll implement these functions using JSON to store and retrieve data.
1. Add Contact Function
The add_contact function will allow us to add new contacts to our system. It takes a Contact object as an argument and appends it to the contacts.json file.
python
CopyEdit
import json
# Function to add a contact
def add_contact(contact, filename=’contacts.json’):
with open(filename, ‘r+’) as file:
data = json.load(file)
data.append(contact.__dict__) # Convert contact object to dictionary
file.seek(0)
json.dump(data, file, indent=4)
2. View All Contacts
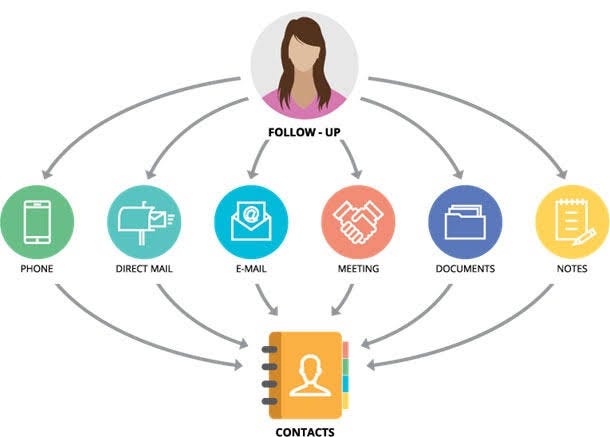
The view_contacts function reads the contacts.json file and displays all stored contacts.
python
CopyEdit
# Function to view all contacts
def view_contacts(filename=’contacts.json’):
with open(filename, ‘r’) as file:
data = json.load(file)
for contact in data:
print(f”Name: {contact[‘name’]}, Phone: {contact[‘phone’]}, Email: {contact[’email’]}”)
3. Delete Contact
The delete_contact function allows you to delete a contact by name. It removes the contact from the contacts.json file.
python
CopyEdit
# Function to delete a contact
def delete_contact(name, filename=’contacts.json’):
with open(filename, ‘r+’) as file:
data = json.load(file)
data = [contact for contact in data if contact[‘name’] != name] # Remove contact by name
file.seek(0)
file.truncate() # Clear the file content before writing new data
json.dump(data, file, indent=4)
4. Search for a Contact
To search for a specific contact by name, we can create a search function. This function will search through the contacts.json file and return the contact if it’s found.
python
CopyEdit
# Function to search a contact by name
def search_contact(name, filename=’contacts.json’):
with open(filename, ‘r’) as file:
data = json.load(file)
for contact in data:
if contact[‘name’].lower() == name.lower():
print(f”Found: Name: {contact[‘name’]}, Phone: {contact[‘phone’]}, Email: {contact[’email’]}”)
return
print(“Contact not found”)
Step 4: Implementing the Menu System
Next, we will implement a menu-driven system that allows users to select which operation they want to perform, such as adding, viewing, deleting, or searching contacts.
python
CopyEdit
def main():
print(“Welcome to the Contact Manager”)
while True:
print(“\nMenu:”)
print(“1. Add Contact”)
print(“2. View Contacts”)
print(“3. Delete Contact”)
print(“4. Search Contact”)
print(“5. Exit”)
choice = input(“Choose an option: “)
if choice == ‘1’:
name = input(“Enter name: “)
phone = input(“Enter phone number: “)
email = input(“Enter email address: “)
contact = Contact(name, phone, email)
add_contact(contact)
print(“Contact added successfully!”)
elif choice == ‘2’:
print(“\nContacts:”)
view_contacts()
elif choice == ‘3’:
name = input(“Enter the name of the contact to delete: “)
delete_contact(name)
print(f”Contact {name} deleted successfully!”)
elif choice == ‘4’:
name = input(“Enter the name of the contact to search: “)
search_contact(name)
elif choice == ‘5’:
print(“Exiting Contact Manager. Goodbye!”)
break
else:
print(“Invalid option, please try again.”)
if __name__ == “__main__”:
main()
Step 5: Testing the Contact Manager
Once the code is set up, you can run the script and test all the functions:
- Add new contacts by providing name, phone, and email.
- View all the stored contacts.
- Delete a specific contact by entering its name.
- Search for a contact by name to see its details.
Expanding Your Contact Manager
At this point, you have a fully functional contact manager. But you can expand it in various ways to improve its functionality. For example:
- Update Contact: Implement functionality to modify existing contact details (such as updating a phone number or email).
- Categorization: Add the ability to categorize contacts (e.g., family, friends, work) and sort contacts by category.
- Search by Multiple Attributes: Extend the search functionality to allow searching by multiple attributes such as phone number or email.
- Graphical User Interface (GUI): Create a more user-friendly experience by building a GUI using libraries like Tkinter or PyQt5.
- Data Export/Import: Allow the ability to import/export contacts to/from CSV files or Excel spreadsheets.
FAQ’s
1. What is the best data storage method for a Python-based contact manager?
The best data storage method depends on your needs. For small projects, using a simple JSON file is a good choice because it is easy to implement and manage. For larger projects with more complex data requirements, you might consider using databases like SQLite, MySQL, or PostgreSQL to handle large volumes of contacts.
2. Can I implement a search feature by multiple attributes in a Python contact manager?
Yes, you can expand the search functionality to filter contacts by multiple attributes, such as name, phone number, and email. This can be achieved by modifying the search_contact function to include multiple search parameters, giving users the flexibility to search based on any combination of contact details.
3. How can I improve the security of the contact manager in Python?
To enhance security, you can implement encryption for contact data stored in the file. Libraries like cryptography can help encrypt sensitive data, such as phone numbers and email addresses, ensuring that the information is protected when stored or transmitted.
4. Can I add a categorization feature for contacts in the Python contact manager?
Yes, you can add a categorization feature to classify contacts into groups such as “Family,” “Friends,” or “Work.” This can be done by adding a new field to the Contact class and modifying the functions to handle and display contacts by category.
5. How can I implement a backup feature for my contact manager in Python?
A backup feature can be implemented by periodically exporting contact data to a separate file or location. You can create a backup function that copies the contents of your contact storage file (e.g., contacts.json) to a backup file, ensuring that your data is not lost in case of accidental deletion or corruption.
Conclusion
Building a contact manager in Python is a great way to improve your programming skills and create a useful application for organizing and managing contacts. With Python’s simple syntax, extensive libraries, and scalability, you can quickly develop a contact manager tailored to your needs. As you build and expand this project, you’ll gain valuable experience in object-oriented programming, file handling, and data management. Whether you use this contact manager for personal use or as a stepping stone to learning more advanced concepts, Python provides a powerful toolset to bring your ideas to life.